Intermediate Examples
This section presents some examples of medium complexity that show how to use μPanel with an Arduino UNO board.
1. Using Sliders
In this example Arduino will read in real-time the position of a Slider on the panel and will update an Analog bar accordingly with 20 Hz of refreshing time.
Hardware
- Arduino UNO Board
- ESP-01 WiFi module (with µPanel Firmware)
- ADP-01 Breadboard adapter
- Breadboard wires (4)
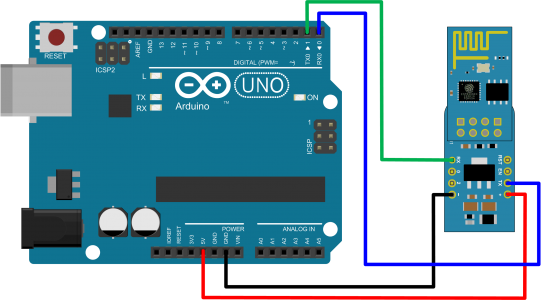
µPanel definition
The panel definition contains the Panel Title, a customised Analog Bar and a Slider. The slider notification interval is set to 50 ms (20 Hz).
D!228;T*15:μPanel-Arduino Example Slider 1;{%100,3!88F,228}//A1%90-3:0:50:100:!F00;/30R1%90:0:100:1:50:50;
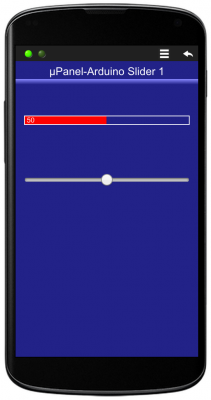
Arduino Code
void setup() { Serial.begin(57600); // Initialise serial delay(3000); // Let's the module start // Send Panel discharging old partial messages Serial.println("\n$P:D!228;T*15:μ-Arduino Example 1;{%100,3!88F,228}//A1%90-3:0:50:100:!F00;/30R1%90:0:100:1:50:50;"); } String Msg; void loop() { int c; while ((c = Serial.read()) > '\n') Msg += (char) c; // Read incoming chars, if any, until new line if (c == '\n') // is message complete? { Msg.toUpperCase(); // Catch partial slider positions too if (Msg.substring(0,4).equals("#R1:")) // has the slider been moved? { Serial.print("#A1:"); // Update the Analog bar Serial.println(Msg.substring(4)); // with the Slider value } Msg = ""; } }
2. Using Sliders (2)
In this example Arduino will read in real-time the position of a Slider on the panel and will update both an Analog bar and a 7-segment based display, with 20 Hz of refreshing time.
Hardware
- Arduino UNO Board
- ESP-01 WiFi module (with µPanel Firmware)
- ADP-01 Breadboard adapter
- Breadboard wires (4)
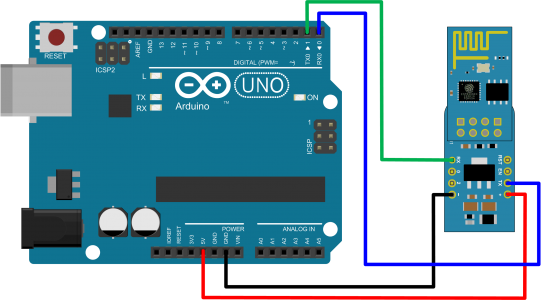
µPanel definition
The panel definition contains the Panel Title, a customised Analog Bar and a Slider. The slider notification interval is set to 50 ms (20 Hz).
D!228;T*15:μPanel-Arduino Example 5;{%100,3!88F,228}//L15:0;L25:0;//A1%90-3:0:50:100:!F00;/30R1%90:0:100:1:50:50;
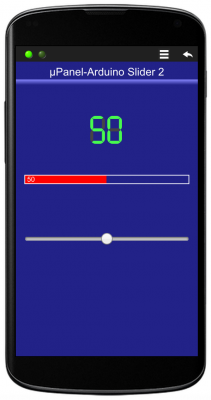
Arduino Code
void setup() { Serial.begin(57600); // Initialise serial delay(3000); // Let's the module start // Send Panel discharging old partial messages Serial.print("\n$P:D!228;T*15:μ-Arduino Slider 2;{%100,3!88F,228}"); Serial.println("//L15:5;L25:0;//A1%90-3:0:50:100:!F00;/30R1%90:0:100:1:50:50;"); } String Msg; void loop() { int c; while ((c = Serial.read()) > '\n') Msg += (char) c; // Read incoming chars, if any, until new line if (c == '\n') // is message complete? { Msg.toUpperCase(); // Catch partial slider positions too if (Msg.substring(0,4).equals("#R1:")) // has the slider been moved? { Serial.print("#A1:"); // Update the Analog bar Serial.println(Msg.substring(4)); // with the Slider value int v = (int) Msg.substring(4).toInt(); // Read the Slider position int d1 = (v / 10) % 10; // Compute the first LCD digit int d2 = v % 10; // Compute the second LCD digit Serial.print("#L1"); Serial.println(d1,DEC); // Update the first LCD digit Serial.print("#L2"); Serial.println(d2,DEC); // Update the second LCD digit } Msg = ""; } }